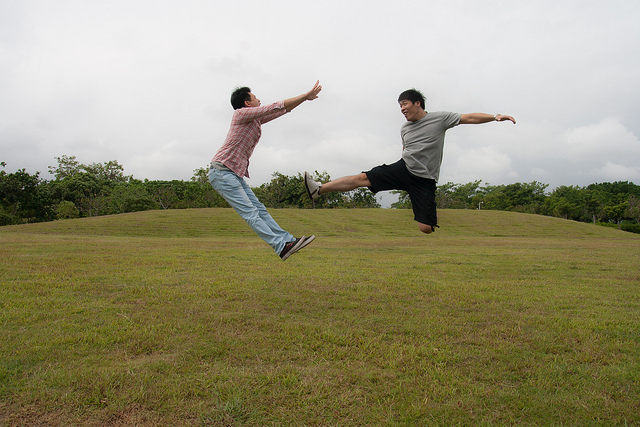
EmguCV Image Process: Transforming Images with Morphological Operations
參考OpenCV 2 Computer Vision Application Programming Cookbook第五章
介紹內容如下:
Eroding and dilating images using morphological filters
Opening and closing images using morphological filters
Detecting edges and corners using morphological filters
Segmenting images using watersheds
Extracting foreground objects with the GrabCut algorithm
上一篇介紹到了兩種常用的形態學運算子(擴張、侵蝕)
在這篇會再介紹兩種,而且和擴張、侵蝕息息相關的運算子:
opening operator(斷開)跟closing operator(閉合)
程式非常的簡單,如下所示:
//Read input image Image<Gray, Byte> image = new Image<Gray, byte>("image.jpg"); //Transform the binary image image._ThresholdBinary(image.GetAverage(), new Gray(255)); //3X3 structuring element StructuringElementEx SElement = new StructuringElementEx(3, 3, 1, 1, Emgu.CV.CvEnum.CV_ELEMENT_SHAPE.CV_SHAPE_RECT); //Applying the opening operator Image<Gray, Byte> opening = image.MorphologyEx(SElement, Emgu.CV.CvEnum.CV_MORPH_OP.CV_MOP_OPEN, 1); //Applying the closing operator Image<Gray, Byte> closing = image.MorphologyEx(SElement, Emgu.CV.CvEnum.CV_MORPH_OP.CV_MOP_CLOSE, 1);利用3X3的structuring element來做運算
使用EmguCV中Image這個類別的MorphologyEx這個方法來處理
可在引數中設定Emgu.CV.CvEnum.CV_MORPH_OP
這是一個列舉,可從中選擇你要的運算子
這裡列出的兩個例子便是:CV_MOP_OPEN跟CV_MOP_CLOSE
處理的影像如下:
正常二值化的影像
閉鎖處理後的影像
斷開處理後的影像
乍看之下,會覺得
斷開跟侵蝕很雷同
閉鎖與侵蝕很雷同
那就來看看原本斷開跟閉鎖的定義:
Closing is defined as the erosion of the dilation of an image.
Opening is defined as the dilation of the erosion of an image.
google的翻譯是:
閉鎖被定義為圖像的擴張的侵蝕
斷開被定義為圖像的侵蝕的擴張
這到底在說什麼!?
直接來看一段程式:
//dilate original image closing = image.Dilate(1); //in-Place erosion of the dilated image closing = closing.Erode(1);先將影像作擴張運算,再做一次侵蝕運算
結果如下:
擴張後侵蝕的處理結果
可以對照一下,跟剛剛使用
image.MorphologyEx(SElement, Emgu.CV.CvEnum.CV_MORPH_OP.CV_MOP_CLOSE, 1)
的結果一模一樣!
所以意思就是:
閉鎖運算 = 先擴張運算 再侵蝕運算
斷開運算 = 先侵蝕運算 再擴張運算
可以透過影像來發現這兩個運算子的特性
經過閉鎖運算:
在白色的影像區塊中,黑色的點會補滿,把白色的區塊做延伸和連結
在黑色的影像區塊中,白色的點會留著
經過斷開運算:
在白色的影像區塊中,黑色的點會留著
在黑色的影像區塊中,白色的點會補滿,把黑色的區塊做延伸和連結
而補滿的狀況,會依據設定的structuring element的大小而有不一樣的結果
要了解到每個運算子的特性
才能活用各個運算子
而在MorphologyEx方法中可以設定執行的次數
可以發現次數越多不見得越有效果
下列是1~6次斷開運算的執行結果:

執行兩次的斷開運算
執行三次的斷開運算
執行四次的斷開運算
執行五次的斷開運算
執行六次的斷開運算
會發現執行次數增加效果會逐漸收斂
這是要特別注意的部分!!
而MorphologyEx內還提供了其他不同的運算子
就留給大家自己試試和研究嘍!!
Black Hat
Top Hat
Morphological Gradient
下一篇介紹透過型態運算來做線段偵測和邊角偵測
沒有留言:
張貼留言